Background
A little while ago I read the following blog post, Web Component for a Code Block by Chris Coyier, it got me thinking about how I could enhance the code blocks on my website. This is a tech blog after all, and from time to time I want to share tips and tricks which involve code snippets. Code syntax highlighting is vital for blog posts because it helps to visually distinguish different elements of code, making it easier to read, understand, and spot errors. HTML has very handy <pre>
and <code>
elements which help with the markup. I previously had some styling that left me with this solution:
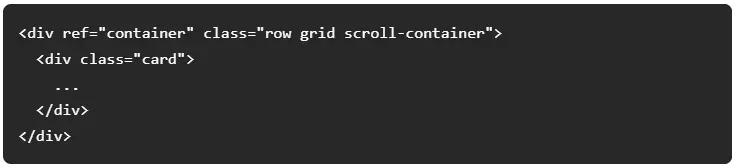
Note that all the text is just white, not at all what you would see in your IDE of choice.
Research & Implementation
All of the content on this blog is hosted on WordPress, and I’ve built a Nuxt module to fetch the content and transform it which I’ll write another blog post on at a later date once I’ve finalised the API for it.
I initially extended my module to process all <pre>
elements in a blog post. I wrote a loop over each element and which let me experiment with a few different syntax highlighting libraries.
Chris had used PrismJS in his web component, so I thought that was as best a place to start as any. It was relatively easy to install with Yarn.
I realised none of my code examples included a language, and Prism needed a language set for each block. I went down a rabbit hole of finding a language detector. Trying to Google that got a lot of results for detecting human languages, such as English or Spanish, rather than code languages, such as JavaScript or PHP. I did find a few detectors, including one based on what GitHub use, Linguist, but most of the auto-detection libraries rely on other contextual clues such as file extension, whereas I just wanted to pass a string to the detector.
I then found a blog from LogRocket, exploring the best syntax highlighting libraries, and looked into Highlight.js. They offer an auto-detection language feature which worked, but wasn’t very accurate. Similar to PrismJS, it was very quick to get a working solution together.
I bit the bullet and went through all of my old blog posts and assigned each code block a language. It didn’t take much longer than 5 minutes as I didn’t have that many code blocks in the first place. I was just over-complicating things!
I stumbled upon another blog post talking about the Torchlight syntax highlighting library and realised that Highlight.js was not very good at Syntax highlighting at all. Torchlight works slightly differently from Highlight.js and PrismJS in that it is a HTTP API. You pass your content to it, and it returns it with syntax highlighting. The highlighter is closed-source, but it uses the engine behind VSCode, so highlighting is much more accurate. The code examples on my site could match exactly what I see in VSCode, perfect! Using Torchlight also meant I could pre-render the highlighting, massively reducing the weight of scripts I was making the end user download. I’m all about performance, so I was sold. There’s a JavaScript client library but it had no docs, so I had to spend a lot of time in the source code figuring out how everything works.
To get started, you import the Torchlight constructor and call the init()
method. The Torchlight constructor takes 3 arguments, a configuration object, a cache class, and a boolean of whether to perform a re-initialisation if Torchlight has already been set up. The library offers a makeConfig()
helper function, which looks for a torchlight.config.js
file in the root directory if it is not passed a configuration object. For my case, I was happy with the defaults Torchlight sets, but wanted to change the theme, so opted to pass this through the function rather than created a config file. I took an informed guess from the options that are available to the other libraries. Torchlight has various themes available which are documented here. Torchlight offers 2 caching mechanisms, a file-based cache, and an in-memory cache. As this site is statically generated, an in-memory cache wouldn’t be any good for me, so I set up a file cache. Again, the library provides a handy helper, allowing you to call new FileCache
or new MemoryCache
. The FileCache
constructor takes a configuration object allowing you to specify the directory in which to cache the files. For each request to the HTTP API, Torchlight stores the response in a JSON file in this directory. When making a request, Torchlight looks in the cache directory first, checking the expiration time, before making a new request to the HTTP API if needed. I omitted the force
argument as this is set to false by default.
1import { makeConfig, torchlight, FileCache } from '@torchlight-api/client' 2 3torchlight.init( 4 await makeConfig({ 5 theme: 'dark-plus' 6 }), 7 new FileCache({ 8 directory: './.torchlight-cache' 9 })10)
I installed the netlify-plugin-cache package which allows you to specify directories that Netlify should cache. This means that the .torchlight-cache
directory persists between builds. I added the following to my netlify.toml
.
1# netlify.toml2[[plugins]]3package = "netlify-plugin-cache"4 [plugins.inputs]5 paths = [6 ".torchlight-cache"7 ]
Now that Torchlight was set up, I actually needed to pass code to it to highlight. There’s another class, block
, which you create for every block of code you want to highlight. You push these into an array and then call torchlight.highlight()
on it, which returns you an array of highlighted blocks. The block
constructor takes 2 arguments, the code, and the language. I’ve added CSS classes to all my code blocks now, so can grab the language from there. I’m using the Cheerio library to parse the WordPress post content, so fetching a class is very simple. I also add the language as a data attribute to the code block, so I can display it with CSS. WordPress’s post content is HTML encoded, so I use the he library to decode it before adding it to the block. This allows HTML inside code blocks to be formatted correctly. The block class generates a unique id, which we set on the <code> element to enable us to update its content once we have received the highlighted code.
1import { load } from 'cheerio' 2import he from 'he' 3import { Block } from '@torchlight-api/client' 4 5$('pre code').each((i, code) => { 6 const $code = $(code) 7 let language = $code.parent().attr('class').split(' ').find((className) => className.startsWith('language-')) || null 8 if (language) { 9 language = language.replace('language-', '')10 $code.parent().attr('data-language', language)11 }12 const torchlightBlock = new Block({13 code: he.decode($code.html()),14 language15 })16 torchlightBlocks.push(torchlightBlock)17 $code.attr('data-torchlight-id', torchlightBlock.id)18})
Now that we have created each block ready for highlighting, we can make the request to the Torchlight API. The JS library has some features to optimise requests, such as sending chunks, so we use the helper function torchlight.highlight()
here. We then loop through each highlighted block and update the HTML with the highlighted version, notice how the ID comes in handy here for selecting the correct code block.
1const highlightedBlocks = await torchlight.highlight(torchlightBlocks)2 3highlightedBlocks.forEach((highlightedBlock) => {4 const $code = $(`pre code[data-torchlight-id="${highlightedBlock.id}"]`)5 6 $code.parent().addClass(highlightedBlock.classes)7 8 $code.html(highlightedBlock.highlighted)9})
That’s it, code snippets have been highlighted in a performant and accurate way. You can see them in action on this very post. I obviously have a lot of custom styling but this is personal preference. The nice thing about Torchlight is that all the styling for the highlighting is done inline, so no need to include any other stylesheets and worry about theming, just change the config property. I do like my implementation of the language identifier though, which you can see a snippet of CSS for below:
1pre.torchlight { 2 position: relative; 3 &:before { 4 position: absolute; 5 top: .75rem; 6 right: 1.25rem; 7 z-index: 10; 8 color: #858585; 9 transition: color .25s ease-out;10 content: attr(data-language)11 }12}
Going back to the original blog post that introduced me to Torchlight, I did want to have a go at adding copy-all functionality to my code snippets. The blog’s example was using Statamic and Alpine, so I had to adapt it to my Nuxt use case. The copyable
Torchlight config option did nothing for me, but the original code was available in the response object under the code
key. The basic idea is to add a container to each code block which contains text to be displayed when a user clicks the copy button, the copy button itself, and the raw code to copy. I could again use the he library to encode the code so that it would display correctly inside of the <code>
element.
1$(` 2<div class="copy-button__container js-copy-to-clipboard-container"> 3 <div class="copy-button__text js-copy-to-clipboard-notification">Copied!</div> 4 <button 5 type="button" 6 title="Copy to clipboard" 7 class="copy-button__button js-copy-to-clipboard-button" 8 > 9 </button>10</div>11<span class="torchlight-copy-target js-copy-to-clipboard-target" style="display: none">${he.encode(highlightedBlock.code)}</span>12`).appendTo($code.parent())
I then wrote some JavaScript for the client side to handle clicking the copy button. I’ve been using classes for this type of thing a lot at Rareloop and it’s really helped me write cleaner code. I’m passing the registerCopyToClipboardContainers()
function to a Vue mixin, but it could be used quite easily in vanilla js.
1class CopyToClipboard { 2 constructor (container) { 3 this.container = container 4 this.notification = this.container.querySelector('.js-copy-to-clipboard-notification') 5 this.button = this.container.querySelector('.js-copy-to-clipboard-button') 6 this.textToBeCopied = this.container.parentElement.querySelector('.js-copy-to-clipboard-target') 7 8 this.button.addEventListener('click', () => { 9 navigator.clipboard.writeText(this.textToBeCopied.innerHTML)10 this.notification.classList.add('copied')11 setTimeout(() => {12 this.notification.classList.remove('copied')13 }, 2000)14 })15 }16}17function registerCopyToClipboardContainers () {18 document.querySelectorAll('.js-copy-to-clipboard-container').forEach((element) => {19 return new CopyToClipboard(element)20 })21}
The CSS is then as simple as below. We hide the raw code, and the copied notification, position the icon at the bottom right of our <pre>
element, and show the copied notification when the .copied
class gets applied.
1.torchlight-copy-target { 2 display: none 3} 4.copy-button__container { 5 position: absolute; 6 right: 1.25rem; 7 bottom: .75rem; 8 z-index: 10; 9 display: flex;10 gap: .5rem;11 align-items: center;12 height: 2rem;13 color: #858585;14 transition: color .25s ease-out;15 &:hover {16 color: white;17 .copy-button__button {18 background-color: white19 }20 }21}22.copy-button__text {23 opacity: 0;24 transition: opacity .25s ease-out;25 &.copied {26 opacity: 127 }28}29.copy-button__button {30 width: 2rem;31 height: 2rem;32 padding: 0;33 background-color: #858585;34 border: 0;35 cursor: pointer;36 mask-image: url("data:image/svg+xml,%3Csvg xmlns='http://www.w3.org/2000/svg' fill='none' viewBox='0 0 24 24' stroke-width='1.5' stroke='currentColor'%3E%3Cpath stroke-linecap='round' stroke-linejoin='round' d='M11.35 3.836c-.065.21-.1.433-.1.664 0 .414.336.75.75.75h4.5a.75.75 0 00.75-.75 2.25 2.25 0 00-.1-.664m-5.8 0A2.251 2.251 0 0113.5 2.25H15c1.012 0 1.867.668 2.15 1.586m-5.8 0c-.376.023-.75.05-1.124.08C9.095 4.01 8.25 4.973 8.25 6.108V8.25m8.9-4.414c.376.023.75.05 1.124.08 1.131.094 1.976 1.057 1.976 2.192V16.5A2.25 2.25 0 0118 18.75h-2.25m-7.5-10.5H4.875c-.621 0-1.125.504-1.125 1.125v11.25c0 .621.504 1.125 1.125 1.125h9.75c.621 0 1.125-.504 1.125-1.125V18.75m-7.5-10.5h6.375c.621 0 1.125.504 1.125 1.125v9.375m-8.25-3l1.5 1.5 3-3.75' /%3E%3C/svg%3E");37 mask-size: contain;38 mask-position: center;39 mask-repeat: no-repeat;40 transition: background-color .25s ease-out41}
The final code for highlighting my post content with Torchlight is below:
1// modules/prepare-wordpress-content/convert-code.js 2import { load } from 'cheerio' 3import he from 'he' 4import { makeConfig, Block, torchlight, FileCache } from '@torchlight-api/client' 5 6export async function syntaxHighlightCodeWithTorchlight (postContent) { 7 const regex = /<pre.*?>/g 8 const matches = postContent.match(regex) 9 if (!matches) {10 return postContent11 }12 const $ = load(postContent, null, false)13 $('pre').removeClass('wp-block-code')14 15 torchlight.init(16 await makeConfig({17 theme: 'dark-plus'18 }),19 new FileCache({20 directory: './.torchlight-cache'21 })22 )23 const torchlightBlocks = []24 25 $('pre code').each((i, code) => {26 const $code = $(code)27 let language = $code.parent().attr('class').split(' ').find((className) => className.startsWith('language-')) || null28 if (language) {29 language = language.replace('language-', '')30 $code.parent().attr('data-language', language)31 }32 const torchlightBlock = new Block({33 code: he.decode($code.html()),34 language35 })36 torchlightBlocks.push(torchlightBlock)37 $code.attr('data-torchlight-id', torchlightBlock.id)38 })39 const highlightedBlocks = await torchlight.highlight(torchlightBlocks)40 41 highlightedBlocks.forEach((highlightedBlock) => {42 const $code = $(`pre code[data-torchlight-id="${highlightedBlock.id}"]`)43 44 $code.parent().addClass(highlightedBlock.classes)45 46 $code.html(highlightedBlock.highlighted)47 48 $(`49 <div class="copy-button__container js-copy-to-clipboard-container">50 <div class="copy-button__text js-copy-to-clipboard-notification">Copied!</div>51 <button52 type="button"53 title="Copy to clipboard"54 class="copy-button__button js-copy-to-clipboard-button"55 >56 </button>57 </div>58 <span class="torchlight-copy-target js-copy-to-clipboard-target" style="display: none">${he.encode(highlightedBlock.code)}</span>59 `).appendTo($code.parent())60 })61 return $.html()62}
For all of the code I added, check out this commit.